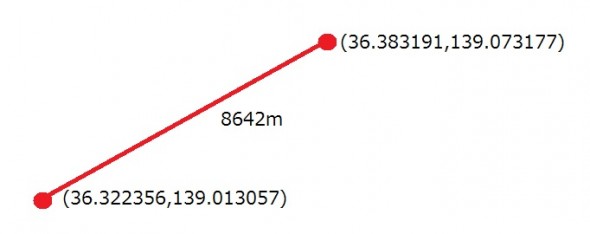
JavaScriptで距離計算など地理情報処理を行うライブラリ「Geolib」
緯度経度のデータから、2点間の距離を求めたり、中心点の算出などが行えるライブラリです。
100%pureJavascriptで書かれているので、node.jsでもフロントエンドでも使用できます。
node.jsでのインストール方法は以下。
1 |
npm install geolib |
サンプル
群馬の地理に詳しくないとよくわからないサンプル。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 |
var geolib = require('geolib'); //2点間の距離を求める var distance = geolib.getDistance( {latitude: 36.322356, longitude: 139.013057}, //高崎駅 {latitude: 36.383191, longitude: 139.073177} //前橋駅 ); console.log(distance+"m"); //->8642m //コンバート console.log(geolib.convertUnit('km', distance) + "km"); //->8.642km console.log(geolib.convertUnit('mi', distance) + "mi"); //->5.3699マイル console.log(geolib.convertUnit('ft', distance) + "ft"); //->28353.0184フィート //中心点を求める var center = geolib.getCenter([ {latitude: 36.322356, longitude: 139.013057}, //高崎駅 {latitude: 36.383191, longitude: 139.073177}, //前橋駅 {latitude: 36.41107600000001, longitude: 139.333037}, //桐生駅 {latitude: 36.294066, longitude: 139.378734}, //太田駅 {latitude: 36.250183, longitude: 139.08331599999997}, //藤岡駅 ]); console.log(center); //->{ latitude: '36.330630', longitude: '139.195896', distance: 37.373 } //含まれるかの判定 var isInside1 = geolib.isPointInside( {latitude: 36.32668100000001, longitude: 139.19382500000006}, //伊勢崎駅 [ {latitude: 36.322356, longitude: 139.013057}, //高崎駅 {latitude: 36.383191, longitude: 139.073177}, //前橋駅 {latitude: 36.41107600000001, longitude: 139.333037}, //桐生駅 {latitude: 36.294066, longitude: 139.378734}, //太田駅 {latitude: 36.250183, longitude: 139.08331599999997}, //藤岡駅 ] ); console.log(isInside1); //->True var isInside2 = geolib.isPointInside( {latitude: 36.490693, longitude: 139.00841100000002}, //渋川駅 [ {latitude: 36.322356, longitude: 139.013057}, //高崎駅 {latitude: 36.383191, longitude: 139.073177}, //前橋駅 {latitude: 36.41107600000001, longitude: 139.333037}, //桐生駅 {latitude: 36.294066, longitude: 139.378734}, //太田駅 {latitude: 36.250183, longitude: 139.08331599999997}, //藤岡駅 ] ); console.log(isInside2); //->False //円内に含まれるかの判定 var inCircle = geolib.isPointInCircle( {latitude: 36.322356, longitude: 139.013057}, //高崎駅 //判別対象 {latitude: 36.383191, longitude: 139.073177}, //前橋駅 //circleの中心点 10000 //半径(m) ); console.log(inCircle); //->True //距離が近い順に並べ替え var distanceList = geolib.orderByDistance( {latitude: 36.322356, longitude: 139.013057}, //高崎駅 [ {latitude: 36.383191, longitude: 139.073177}, //前橋駅 {latitude: 36.41107600000001, longitude: 139.333037}, //桐生駅 {latitude: 36.294066, longitude: 139.378734}, //太田駅 {latitude: 36.250183, longitude: 139.08331599999997}, //藤岡駅 ] ); console.log(distanceList); /* -> [ { key: '0', latitude: 36.383191, //前橋駅 longitude: 139.073177 distance: 8642 }, { key: '3', latitude: 36.250183, //藤岡駅 longitude: 139.083315 distance: 10197 }, { key: '1', latitude: 36.41107600 //桐生駅 longitude: 139.333037 distance: 30357 }, { key: '2', latitude: 36.294066, //太田駅 longitude: 139.378734 distance: 32992 } ] */ //一番近いポイントを抽出 var near = geolib.findNearest( {latitude: 36.322356, longitude: 139.013057}, //高崎駅 [ {latitude: 36.383191, longitude: 139.073177}, //前橋駅 {latitude: 36.41107600000001, longitude: 139.333037}, //桐生駅 {latitude: 36.294066, longitude: 139.378734}, //太田駅 {latitude: 36.250183, longitude: 139.08331599999997}, //藤岡駅 ] ); console.log(near); //-> { key: '0', latitude: 36.383191, longitude: 139.073177, distance: 8642 } //前橋駅 |