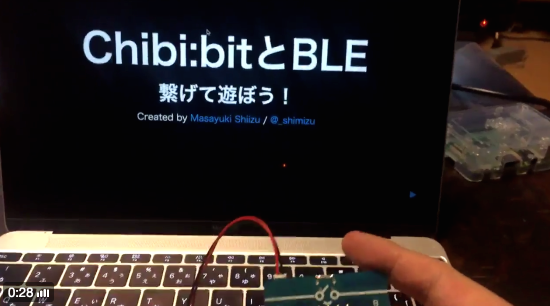
Reveal.jsをMicro:bit(chibi:bit)でコントロールする。
Reveal.jsで作成したスライドをchibi:bitで操作してみます。
"GitHub – shimizu/microbit-ble-example" microbit(chibibit)でスライド操作するサンプルコードをgithubに上げた。https://t.co/KVBWXZmtnA pic.twitter.com/G8PpjhaXap
— 清水正行 (@_shimizu) February 1, 2017
仕組み
chibi:bitとMacをBLEで繋ぎボタン操作を取得します。それをwebsocketを通じてReveal.jsに伝達することで、スライドのページめくりを実装しています。
サンプルコードは下記リポジトリにアップしてあります。
shimizu/microbit-ble-example: node.jsでmicrobitをBLEを使って操作するサンプル
node-bbc-microbit
node.jsライブラリ「node-bbc-microbit」を使用することで、chibi:bit(micro:bit)をBLEでパソコンにつなぐことができます。
node-bbc-microbitの使い方については、READMEに掲載されているのでそちらを参照してください。
sandeepmistry/node-bbc-microbit: Control a BBC micro:bit from Node.js using BLE
ボタン操作を取得するサンプル
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
var BBCMicrobit = require('bbc-microbit') var BUTTON_VALUE_MAPPER = ['Not Pressed', 'Pressed', 'Long Press']; console.log('chibi:bitを検索しています') BBCMicrobit.discover(function(microbit) { console.log('chibi:bitを発見しました: id = %s, address = %s', microbit.id, microbit.address) microbit.on('disconnect', function() { console.log('エラー!:接続できませんでした') process.exit(0) }) microbit.connectAndSetUp(function() { console.log('chibi:bitに接続しました') microbit.subscribeButtons(function() { console.log('ボタン入力を受付中') }) }) microbit.on('buttonAChange', function(value) { console.log('Aボタン: ', BUTTON_VALUE_MAPPER[value]) }) microbit.on('buttonBChange', function(value) { console.log('Bボタン: ', BUTTON_VALUE_MAPPER[value]) }) }) |
スライド操作サンプル
Reveal.jsで作成したスライドを表示するために、webサーバーとして「serve-static」モジュールを使用しています。websocketサーバーは「socket.io」を使用。
BLEでは、非同期処理がどうしても多くなるのでpromisでまとめました。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 |
var BBCMicrobit = require('bbc-microbit') var exec = require('child_process').exec var http = require('http') var finalhandler = require('finalhandler') var serveStatic = require('serve-static') var BUTTON_VALUE_MAPPER = ['Not Pressed', 'Pressed', 'Long Press']; console.log('chibi:bitを検索しています') var discoverMcrobit = new Promise(function(resolve, reject){ BBCMicrobit.discover(function(microbit) { console.log('\tchibi:bitを発見しました: id = %s, address = %s', microbit.id, microbit.address) microbit.on('disconnect', function() { console.log('\t接続エラー!:chibi:bitに接続できませんでした') process.exit(0); }) resolve(microbit) }) }) var subscribeButtons = function(microbit){ return new Promise(function(resolve, reject){ microbit.connectAndSetUp(function() { console.log('\t接続しました') microbit.subscribeButtons(function() { console.log('\tボタン入力を受付中'); resolve(microbit) }) }) }) } var onClickButtons = function(collection){ var microbit = collection.microbit var socket = collection.socket return new Promise(function(resolve, reject){ microbit.on('buttonAChange', function(value) { console.log('\ton -> button A change: ', BUTTON_VALUE_MAPPER[value]); if (BUTTON_VALUE_MAPPER[value] == "Pressed") socket.emit('greeting', {message: 'A button'}) }) microbit.on('buttonBChange', function(value) { console.log('\ton -> button B change: ', BUTTON_VALUE_MAPPER[value]); if (BUTTON_VALUE_MAPPER[value] == "Pressed") socket.emit('greeting', {message: 'B button'}) }) resolve(microbit) }) } function runWebscoketServer(microbit) { return new Promise(function(resolve, reject){ var io = require('socket.io').listen(8124); exec("open http://localhost:8000") io.sockets.on('connection', function(socket) { resolve({microbit:microbit, socket:socket}) }) }) } //webserver 起動 var serve = serveStatic(__dirname, {'index': ['index.html', 'index.htm']}) var server = http.createServer(function onRequest (req, res) { serve(req, res, finalhandler(req, res)) }) server.listen(8000) discoverMcrobit.then(subscribeButtons).then(runWebscoketServer).then(onClickButtons) |