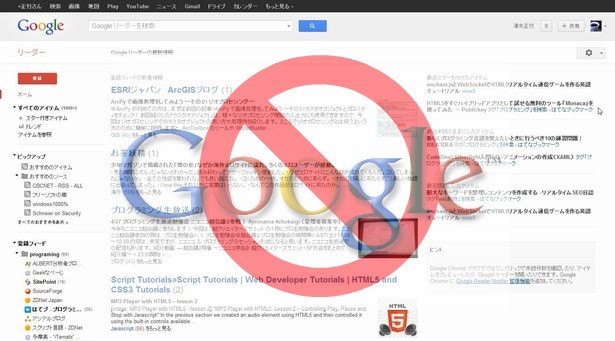
【node.js+D3.js】RSSリーダーを作る
feedparserの仕様が変わったため現在動きません。
Googleが酷いことをしたので自前のRSSリーダーを作成してみます。
フィードを読み込んでリストとして表示するだけの、最低限の機能しかありません。
node.jsでRSS取得サーバーを作成
フィードの読み込みには「feedparser」ライブラリを使用します。
1 |
$ npm install feedparser |
feedパラメーターにフィードのURLを指定してGETリクエストを送ると取得した内容をJSONで返すだけのサーバーです。(WEB API)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
var FeedParser = require('feedparser') , request = require('request') , fs = require('fs') , http = require('http') , url = require('url'); http.createServer(function (req, res) { var pathname = url.parse(req.url).pathname; var queryData = url.parse(req.url, true).query; var feedurl = unescape(queryData.feed) var article = []; if(pathname === "/favicon.ico") return; request(feedurl) .pipe(new FeedParser()) .on('error', function(error) { console.error(error); }) .on('meta', function (meta) { //console.error(meta); }) .on('readable', function () { // do something else, then do the next thing var stream = this, item; while (item = stream.read()) { article.push(item); } stream.end = function(){ res.writeHead(200, { 'Content-Type':'application/json; charset=utf-8', 'Access-Control-Allow-Origin':'*', 'Pragma': 'no-cache', 'Cache-Control' : 'no-cache' }); if(queryData.limit) article = article.slice(0, queryData.limit); res.write(JSON.stringify(article)); res.end(); }; }); process.on('uncaughtException', function (err) { console.log('uncaughtException => ' + err); console.log(feedurl); res.writeHead(500); res.write("error!"); res.end(); }); }).listen(1337, '127.0.0.1'); function send(){ } console.log('Server running at http://127.0.0.1:1337/?feed=<RSS URL>&limit=<length>'); |
ローカルで動かすこと前提なので、セキュリティとか考慮してません。
D3.jsでRSSリーダーのフロントエンドを作成
D3.jsはグラフの作成だけでなく、フロントエンドのUIを作成するのにも使えます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <script src="http://d3js.org/d3.v3.min.js"></script> <script> var list = [ //読み込むフィードの指定 {title:"GUNMA GIS GEEK", url:"https://gunmagisgeek.com/blog/feed", limit:5}, {title:"はてブ", url:"http://feeds.feedburner.com/hatena/b/hotentry", limit:5}, {title:"WIRED.jp", url:"http://wiredvision.jp/feed/atom.xml", limit:5} ] //IE v.9以下非対応 list.map(function(d){ d.id = d.title.replace(/s|t| |.|,|\/g,""); //titleからDOM idを作成 }); var date = new Date(); window.onload = function(){ var ul = d3.select('body').selectAll('ul').data(list) .enter() .append('div') .attr('id', function(d){ return d.id}) .append('ul') ul.html(function(d){ return "<h3>" + d.title + "</h3>"}) .attr('class', function(d){ return d.id + "_ul"}) .selectAll(append_li) function append_li(d){ var date = new Date(); var encUrl = escape(d.url); d3.json('http://127.0.0.1:1337/?feed='+encUrl+'&limit='+d.limit + "&"+date, function(json){ d3.select('.'+d.id +"_ul") .selectAll("."+d.id+"_list") .data(json) .enter() .append('li') .attr("class", d.id+"_list") .html(function(d){ return '<a href="'+d.link+'" target="_blank">' + d.title + '</a>'; }) }); return 'li'; } } </script> </head> <body> </body> </html> |
実行
まずサーバーを起動します。
1 |
node server.js |
サーバーが起動したら、reader.htmlを開きます。以上。
https://github.com/shimizu/SimpleRssReader